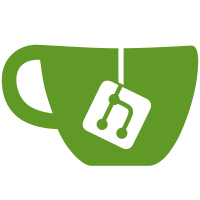
* Unit tests for refactoring Spreadsheet properties * Refactor Xlsx Properties Reader code into a separate class
92 lines
3.4 KiB
PHP
92 lines
3.4 KiB
PHP
<?php
|
|
|
|
namespace PhpOffice\PhpSpreadsheetTests\Reader;
|
|
|
|
use PhpOffice\PhpSpreadsheet\Document\Properties;
|
|
use PhpOffice\PhpSpreadsheet\Reader\Xlsx;
|
|
use PhpOffice\PhpSpreadsheet\Shared\File;
|
|
use PHPUnit\Framework\TestCase;
|
|
|
|
class XlsxTest extends TestCase
|
|
{
|
|
public function testLoadWorkbookProperties()
|
|
{
|
|
$filename = './data/Reader/XLSX/propertyTest.xlsx';
|
|
$reader = new Xlsx();
|
|
$spreadsheet = $reader->load($filename);
|
|
|
|
$properties = $spreadsheet->getProperties();
|
|
// Core Properties
|
|
$this->assertEquals('Mark Baker', $properties->getCreator());
|
|
$this->assertEquals('Unit Testing', $properties->getTitle());
|
|
$this->assertEquals('Property Test', $properties->getSubject());
|
|
// Extended Properties
|
|
$this->assertEquals('PHPOffice', $properties->getCompany());
|
|
$this->assertEquals('The Big Boss', $properties->getManager());
|
|
// Custom Properties
|
|
$customProperties = $properties->getCustomProperties();
|
|
$this->assertInternalType('array', $customProperties);
|
|
$customProperties = array_flip($customProperties);
|
|
$this->assertArrayHasKey('Publisher', $customProperties);
|
|
$this->assertTrue($properties->isCustomPropertySet('Publisher'));
|
|
$this->assertEquals(Properties::PROPERTY_TYPE_STRING, $properties->getCustomPropertyType('Publisher'));
|
|
$this->assertEquals('PHPOffice Suite', $properties->getCustomPropertyValue('Publisher'));
|
|
}
|
|
|
|
/**
|
|
* Test load Xlsx file without cell reference.
|
|
*/
|
|
public function testLoadXlsxWithoutCellReference()
|
|
{
|
|
$filename = './data/Reader/XLSX/without_cell_reference.xlsx';
|
|
$reader = new Xlsx();
|
|
$reader->load($filename);
|
|
}
|
|
|
|
/**
|
|
* Test load Xlsx file and use a read filter.
|
|
*/
|
|
public function testLoadWithReadFilter()
|
|
{
|
|
$filename = './data/Reader/XLSX/without_cell_reference.xlsx';
|
|
$reader = new Xlsx();
|
|
$reader->setReadFilter(new OddColumnReadFilter());
|
|
$data = $reader->load($filename)->getActiveSheet()->toArray();
|
|
$ref = [1.0, null, 3.0, null, 5.0, null, 7.0, null, 9.0, null];
|
|
|
|
for ($i = 0; $i < 10; ++$i) {
|
|
$this->assertEquals($ref, \array_slice($data[$i], 0, 10, true));
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Test load Xlsx file with drawing having double attributes.
|
|
*/
|
|
public function testLoadXlsxWithDoubleAttrDrawing()
|
|
{
|
|
if (version_compare(PHP_VERSION, '7.0.0', '<')) {
|
|
$this->markTestSkipped('Only handled in PHP version >= 7.0.0');
|
|
}
|
|
$filename = './data/Reader/XLSX/double_attr_drawing.xlsx';
|
|
$reader = new Xlsx();
|
|
$reader->load($filename);
|
|
}
|
|
|
|
/**
|
|
* Test correct save and load xlsx files with empty drawings.
|
|
* Such files can be generated by Google Sheets.
|
|
*/
|
|
public function testLoadSaveWithEmptyDrawings()
|
|
{
|
|
$filename = __DIR__ . '/../../data/Reader/XLSX/empty_drawing.xlsx';
|
|
$reader = new Xlsx();
|
|
$excel = $reader->load($filename);
|
|
$resultFilename = tempnam(File::sysGetTempDir(), 'phpspreadsheet-test');
|
|
$writer = new \PhpOffice\PhpSpreadsheet\Writer\Xlsx($excel);
|
|
$writer->save($resultFilename);
|
|
$excel = $reader->load($resultFilename);
|
|
// Fake assert. The only thing we need is to ensure the file is loaded without exception
|
|
$this->assertNotNull($excel);
|
|
}
|
|
}
|